Unlock the full power of your mobile and edge devices
Automatically communicate Peer-to-Peer
Devices embedded with the Ditto Edge SDK automatically create wireless, mobile mesh networks to enable real-time data sharing in bandwidth-constrained environments.
We utilize multiple connection technologies including Bluetooth Low Energy, Peer-to-Peer Wi-Fi, and Local Area Network to reliably sync data without the internet and without unnecessary network hardware.
Automatic device discovery and mesh creation
Developer friendly and platform-agnostic
Sync up to 130 m / 425 ft and even further with multihop
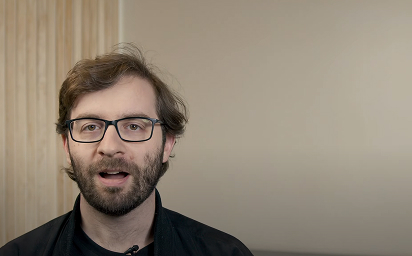
Ditto Rainbow Connection
Automatic Peer-to-Peer Over All Available Transfers
By default, Ditto maintains multiple offline transports and automatically switches to the fastest transport - without duplicating data - based on distance and strength of connection.

The Edge SDK always find a path to sync your data by utilizing Multihop sync
Multihop sync is the process of passing data from one connected peer to another by the way of intermediate “hops”. Multihop allows for fast, long-range data transfer without internet reliance.
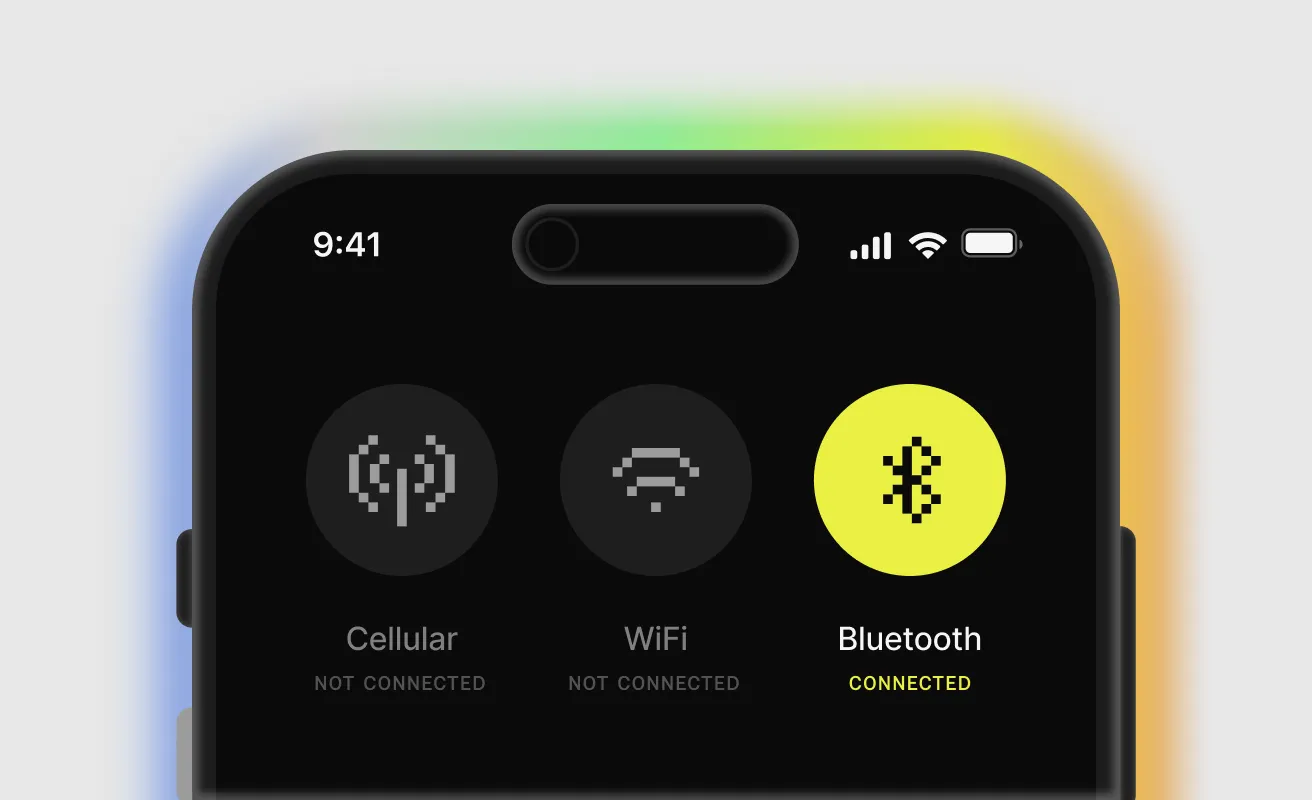
Operational efficiency when disconnected
Equipped with an embedded database, the Edge SDK enables devices to read and write data when disconnected from the internet, ensuring local data sharing and access at all times.

Sync cross-platform, conflict-free
The Edge SDK is platform agnostic across iOS, Android, Windows, and Linux. Automatically resolve online and offline conflicts between these peers and with the cloud when available.
Harness the full power of your existing mobile and edge infrastructure
More WiFi isn’t the answer. Your mobile and edge devices are more powerful than you realize, capable of advanced networking without the need for new devices, hardware, or networking setups.
Our APIs embrace reactive principles with excellent support for Flutter, React, SwiftUI, Jetpack Compose, and more.